Here is an example of a basic player controller script that can be used to move a player character in a 3D game:
using UnityEngine;
public class PlayerController : MonoBehaviour
{
public float moveSpeed = 5f;
public float jumpForce = 5f;
public bool isGrounded;
public bool canJump;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void Update()
{
float moveX = Input.GetAxis(“Horizontal”);
float moveZ = Input.GetAxis(“Vertical”);
Vector3 movement = new Vector3(moveX, 0f, moveZ);
rb.AddForce(movement * moveSpeed);
if (Input.GetButtonDown(“Jump”) && isGrounded)
{
rb.AddForce(new Vector3(0f, jumpForce, 0f), ForceMode.Impulse);
isGrounded = false;
}
}
void OnCollisionEnter(Collision collision)
{
if (collision.gameObject.CompareTag(“Ground”))
{
isGrounded = true;
}
}
}
This script uses the Input.GetAxis() function to get the horizontal and vertical input from the player, and then applies a force to the Rigidbody component to move the player character. It also has a basic jumping functionality that checks if the player is on the ground and if the jump button is pressed, it applies an upward force to the Rigidbody. The script also uses the OnCollisionEnter() function to check if the player character is colliding with the ground and if so, it sets the isGrounded variable to true.
Please note that this is a basic example, you can add many features to it, like camera control, animations, sound effects, and much more, also, you should customize it to your specific needs and game mechanics.
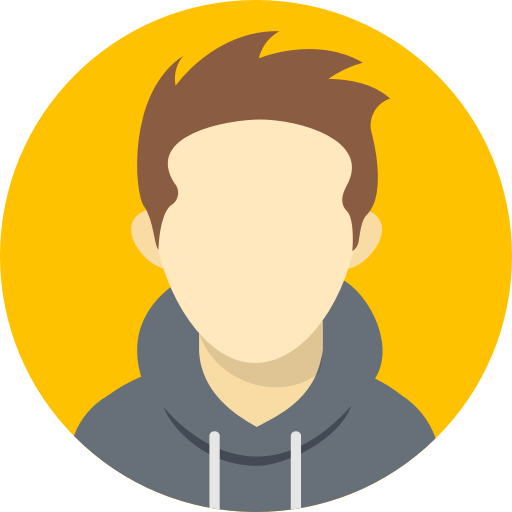
Analyst studies emerging trends in the information technology.