using UnityEngine;
public class PlatformGenerator : MonoBehaviour
{
public GameObject platformPrefab;
public float platformWidth = 5f;
public float platformHeight = 1f;
public float platformDepth = 5f;
public float platformSpawnRadius = 10f;
private void Start()
{
Vector3 platformPosition = Random.onUnitSphere * platformSpawnRadius;
platformPosition.y = 0f;
platformPosition += transform.position;
Vector3 platformScale = new Vector3(
Random.Range(0.5f, 2f) * platformWidth,
platformHeight,
Random.Range(0.5f, 2f) * platformDepth
);
GameObject platform = Instantiate(platformPrefab, platformPosition, Quaternion.identity);
platform.transform.localScale = platformScale;
}
}
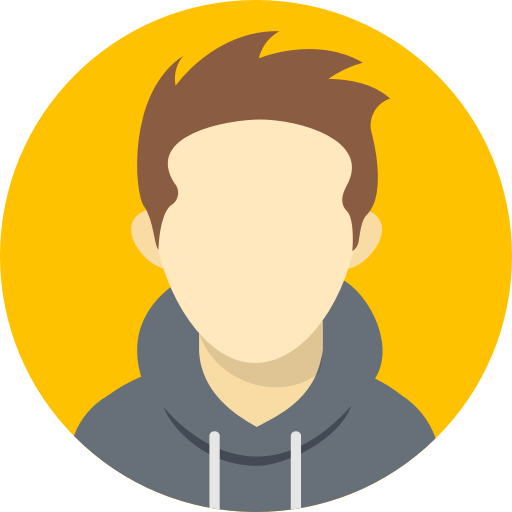
Analyst studies emerging trends in the information technology.