using UnityEngine;
public class ObjectMoveAlongPath : MonoBehaviour
{
public Transform[] path;
public float speed = 2f;
private int currentPathIndex = 0;
private Vector3 currentTarget;
private void Start()
{
currentTarget = path[0].position;
}
private void Update()
{
if (Input.GetKey(KeyCode.UpArrow))
{
transform.position = Vector3.MoveTowards(transform.position, currentTarget, speed * Time.deltaTime);
if (Vector3.Distance(transform.position, currentTarget) < 0.1f)
{
currentPathIndex = (currentPathIndex + 1) % path.Length;
currentTarget = path[currentPathIndex].position;
}
}
if (Input.GetKey(KeyCode.DownArrow))
{
transform.position = Vector3.MoveTowards(transform.position, currentTarget, -speed * Time.deltaTime);
if (Vector3.Distance(transform.position, currentTarget) < 0.1f)
{
currentPathIndex = (currentPathIndex + path.Length - 1) % path.Length;
currentTarget = path[currentPathIndex].position;
}
}
}
}
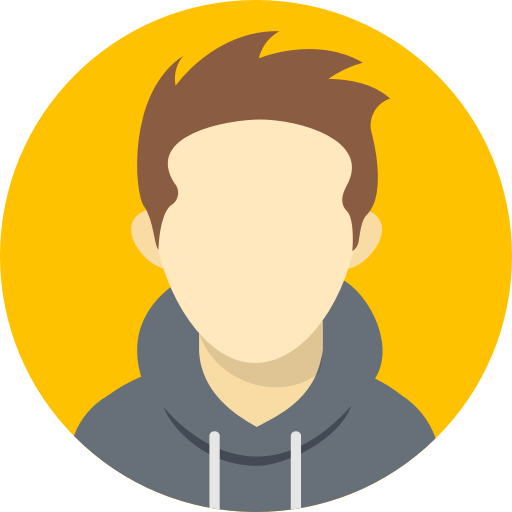
Analyst studies emerging trends in the information technology.